Modern Java: Why You Might Not Need Lombok Anymore
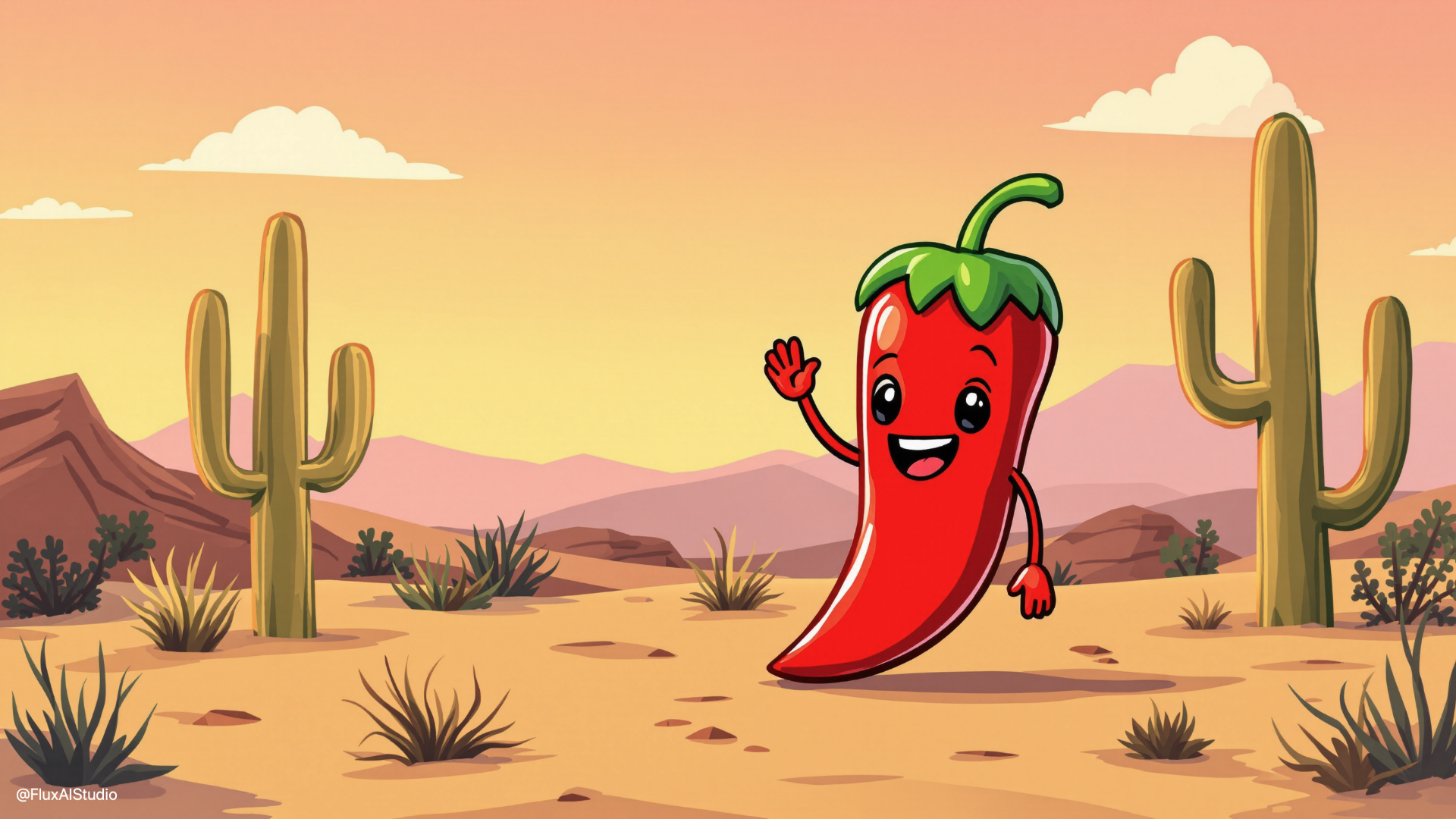
If you've been developing Java applications over the past decade, you've likely encountered or used Project Lombok. This library promised to eliminate boilerplate code through annotations, making Java development more concise and enjoyable. However, as Java has evolved and modern development practices have emerged, it's time to reevaluate Lombok's place in our toolbox.
The Hidden Costs of Lombok
While Lombok's promise of reducing boilerplate code is appealing, it comes with significant drawbacks that aren't immediately apparent. Recent discussions in the Java community have highlighted serious concerns about Lombok's impact on development workflows and tool chains. Let's examine why many developers and teams are moving away from it.
Breaking IDE Features
One of the most frustrating aspects of Lombok is its interference with IDE functionality. Consider this scenario:
@Builder
public class OrderService {
private final OrderRepository repository;
// ... service methods
}
When you try to find all usages of this class's builder (using OrderService.builder()
), your IDE's "Find Usages" feature may fail to locate all instances. This breaks one of the most fundamental features developers rely on for code navigation and refactoring. As codebases grow, this limitation becomes increasingly problematic for maintenance and code reviews.
Annotation Processor Conflicts
Lombok's aggressive manipulation of the compilation process often conflicts with other annotation processors. This isn't just an inconvenience—it can prevent you from using important tools in your development stack:
- MapStruct for object mapping
- QueryDSL for type-safe queries
- JPA Buddy for entity management
- Other custom annotation processors in your toolchain
The root cause? Lombok doesn't play by the rules of standard annotation processing. Instead, it hijacks the compilation process, leading to unpredictable interactions with other processors.
Debugging and Development Challenges
One of the most significant issues with Lombok is its impact on debugging. Since Lombok modifies the Abstract Syntax Tree (AST) at compile time, the code you write isn't the code that runs. This can lead to several problems:
@Data
public class Customer {
private String name;
private List<Order> orders;
}
This seemingly simple class hides generated methods that you can't step through during debugging. When issues arise, you're left investigating code that doesn't exist in your source files.
IDE Integration and Tooling Issues
Modern IDEs are powerful development tools, but Lombok can interfere with their functionality:
- Find usages features become unreliable
- Code navigation breaks down
- Refactoring tools may not work as expected
- Auto-completion can be inconsistent
AST Manipulation and Compilation Issues
Lombok's approach to code generation is fundamentally different from standard annotation processors. It directly manipulates the Abstract Syntax Tree (AST) during compilation, which leads to several serious issues:
@Data
@Builder
public class Customer {
private String name;
private List<Order> orders;
// Imagine dozens of fields and complex relationships
}
When this code compiles:
- Lombok modifies the AST before other processors see it
- This breaks incremental compilation, forcing full recompilation more often
- The generated code isn't visible until after compilation
- The actual bytecode might not match what you expect
The impact on build times can be significant, especially in larger projects. What's worse, these issues compound as your project grows.
Debugger Alterations and Java Version Compatibility
Two of the most serious issues with Lombok affect core development workflows:
- Debugger Interference:
- You can't step into generated methods
- Breakpoints in generated code don't work as expected
- Stack traces can be confusing or misleading
- Java Version Compatibility:
// This might work in Java 17... @Data public class Product { private final BigDecimal price; } // But break in Java 21 due to Lombok's use of internal APIs
Lombok relies on internal compiler APIs (com.sun.tools.javac
), which:
- Are not part of the public API
- Can change without warning
- Often break with new Java releases
- Force teams to wait for Lombok updates before upgrading Java versions
Modern Java Solutions
The good news is that modern Java provides better alternatives to Lombok. These solutions don't just avoid Lombok's problems—they often provide better, more maintainable approaches that work reliably with all development tools. Here's how you can achieve the same goals with standard Java features:
Records for Data Classes
Instead of using @Data
, leverage Java records:
// Before with Lombok
@Data
public class Product {
private final String name;
private final BigDecimal price;
}
// After with Java Record
public record Product(String name, BigDecimal price) {
}
Records provide immutability, proper equals/hashCode implementations, and a clear indication of the class's purpose.
Builder Pattern Without Lombok
While Lombok's @Builder
is convenient, modern IDEs can generate builder patterns with a few clicks:
public class Order {
private final String id;
private final LocalDateTime createdAt;
private final List<Product> products;
private Order(Builder builder) {
this.id = builder.id;
this.createdAt = builder.createdAt;
this.products = List.copyOf(builder.products);
}
public static Builder builder() {
return new Builder();
}
// Builder class with fluent API
public static class Builder {
private String id;
private LocalDateTime createdAt;
private List<Product> products = new ArrayList<>();
public Builder id(String id) {
this.id = id;
return this;
}
// Additional builder methods...
public Order build() {
return new Order(this);
}
}
}
Migration Strategy
If you're convinced it's time to move away from Lombok, here's a practical approach to migration:
- Start with new code:
- Use records for simple data classes
- Leverage IDE generation for constructors and methods
- Document standard patterns for the team
- Gradual migration of existing code:
- Identify classes with minimal Lombok usage
- Convert one annotation at a time
- Use IDE refactoring tools to generate standard Java code
- Add tests before making changes
- Tooling support:
- Configure IDE templates for common patterns
- Set up code style guidelines
- Use static analysis tools to enforce practices
The Real Cost of Convenience
The Java community's initial embrace of Lombok was understandable—Java was verbose, and Lombok offered a seductive solution. However, we now know this convenience came at a steep price:
- Broken IDE features that hamper productivity
- Unreliable debugging that costs development time
- Build tool conflicts that complicate project setup
- Java version upgrades that become more difficult
- Development tools that can't be fully utilized
Conclusion
While Lombok served a purpose in Java's history, its costs now far outweigh its benefits. The introduction of records, enhanced IDE capabilities, and modern Java features provide better solutions without the drawbacks of bytecode manipulation and tooling interference.
The path forward is clear: embrace modern Java features, leverage your IDE's capabilities, and gradually migrate away from Lombok. Your future self (and your team) will thank you for having:
- Fully debuggable code
- Reliable IDE features
- Faster builds with proper incremental compilation
- Easier Java version upgrades
- Better integration with the entire Java ecosystem
Remember, good code isn't just about reducing verbosity—it's about clarity, maintainability, and leveraging the best tools for the job. As our tools and language evolve, so should our practices.
Happy coding! 🚀
P.S. If you're starting a new project, save yourself future headaches and skip Lombok entirely. Your development tools will thank you.